How Does React Work?
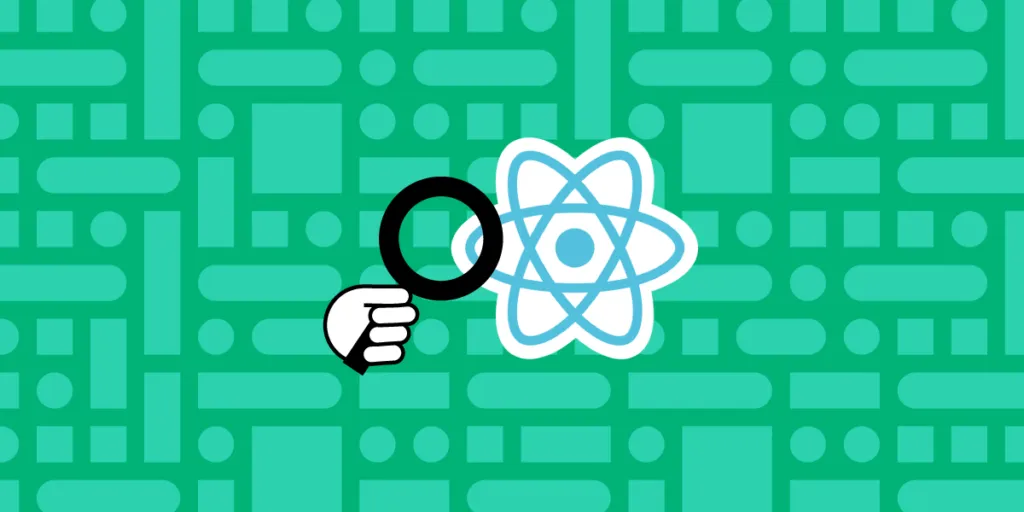
React is a JavaScript library used for building user interfaces, especially for single-page applications where the user interacts with the web page without requiring a full page reload. Developed and maintained by Facebook, React has become popular for its declarative approach to building UI components and its efficient rendering through a virtual DOM (Document Object Model).
Build React app prototypes that are ready for development with one click. Drag-and-drop UI components that come from best open-source React libraries like MUI, Ant design, React Bootstrap or bring in your own React components. Try UXPin Merge.
What is React?
React, also known as ReactJS or React.js, is a JavaScript library for front-end development. It was originally developed by Facebook and is now maintained by Facebook and Instagram developers, along with contributions from the open-source community.
React is widely used for creating interactive and dynamic web applications and websites, and it has been employed in the development of some major websites and apps such as PayPal and Netflix.
React simplifies the process of building user interfaces by offering a declarative and component-based approach, leveraging the power of JavaScript, and optimizing performance through features like the virtual DOM.
It’s often confused with React Native — a JavaScript framework, whereas React is commonly referred to React js — a React library. Learn about the differences between React js and React Native here.
What is React often compared with?
React is often compared with other JavaScript libraries and frameworks, such as Angular, Vue or Svelte.
Angular is a JavaScript framework. While both are used for building dynamic web applications, React is a library focused on the view layer, providing more flexibility in terms of integrating with other tools. Angular, on the other hand, is a comprehensive framework that comes with an opinionated structure and a set of tools for building large-scale applications.
When it comes to Vue, it is a progressive JavaScript framework that shares similarities with React in terms of being component-based. Vue.js is often considered more approachable for beginners due to its simplicity, while React is favored for its flexibility and a larger ecosystem of libraries and tools.
Lastly, Svelte is a newer approach to web development that shifts much of the work from the browser to the build step. Unlike React, which works with a virtual DOM, Svelte shifts the work to compile time, resulting in smaller, more efficient code at runtime. React’s virtual DOM approach is often contrasted with Svelte’s compile-time approach.
How does React work?
React works by combining several key principles and features that contribute to its efficiency and flexibility in building user interfaces.
An overview of how React works
Here’s an overview of how it works:
- Declarative views — React adopts a declarative approach, where developers describe how the user interface should look based on different states and data. When the underlying data changes, React efficiently updates and renders only the components that are affected, simplifying the development process and enhancing the user experience.
- JavaScript code and JSX — React is written in JavaScript, one of the most widely used programming languages. JSX, a syntax extension for JavaScript, allows developers to write UI components in a format that resembles XML or HTML. This makes the code more readable and expressive, contributing to a more efficient development workflow.
- Component-based architecture — React applications are structured using a component-based architecture. Components are modular, self-contained units of code that represent different parts of the user interface. This modularity promotes code reusability, making it easier to manage and maintain large codebases. React has functional components and class components.
- Hierarchical arrangement of components — One of the key advantages of this architecture lies in the relationship between parent and children components. In React, components can be arranged hierarchically, with some components serving as parents and others as children. The parent component encapsulates the logic or functionality that is common to its children, promoting a structured and organized codebase.
- Virtual DOM — React uses a virtual DOM (short for Document Object Model) to optimize the manipulation of the actual DOM. Instead of directly updating the entire DOM when data changes, React first creates a virtual representation of the DOM in memory. It then calculates the most efficient way to update the actual DOM, reducing the need for full page reloads and improving performance.
- JavaScript libraries integration — React’s open-source nature and popularity make it compatible with a variety of JavaScript libraries. These libraries, developed by the community, offer pre-written code for various functionalities. Integrating these libraries into React applications helps save development time and effort, allowing developers to leverage existing solutions. Here you can find examples of those libraries based on their popularity.
How to make React work
React developers usually set up a React project to make it work. The process consists of several steps that provide a basic setup for a React project. First, they install Node.js and npm. After this, they create React app. They open a terminal or command prompt and use the create-react-app command to create a new React app. This command creates a new directory called my-react-app
with the basic structure of a React app.
Try this yourself if you want to learn React. Install Node.js and npm with this command:
npx create-react-app my-react-app
Then, move into the newly created project directory with this:
cd my-react-app
Start the development server to preview your app locally by typing in:
npm start
Familiarize yourself with the project structure. Key directories include src
(source code), public
(static assets), and various configuration files such as package.json
and src/index.js
. React applications are built using components. Open the src/App.js
file to see the default component. JSX, a syntax extension for JavaScript, is used to define the component structure.
If you need more resources, check out this simple article: Create your first React app.
Instead of adding or editing components in code, you can use a UI builder like UXPin Merge to build a React app’s user interface and copy the code from the design directly to Stackblitz or other dev environment to set up data structures and deploy your React project.
UXPin has built-in React component libraries, such as MUI, Bootstrap or Ant design and it works by dropping the components on the canvas to arrange an app layout. You can build any layout you want with React elements that are on your disposal, be it an employee portal or a podcast app. And you can bring in your own library of React components if you have one. The components are fully customizable and functional, so you can see how your app would work before deployment. Try UXPin Merge for free.
Why use React?
React, a powerful JavaScript library, offers a multitude of compelling reasons for its widespread adoption in the development community.
- Open Source — React is an open-source library, maintained by both Facebook and Instagram developers, along with a large and active community. This community contributes to the ongoing improvement of React, develops additional libraries (e.g., Redux for state management), and provides support through forums and documentation.
- Individual component editing — React follows a downward data flow, meaning that changes in a component do not impact components higher in the hierarchy. This enables developers to edit and update individual components without affecting the entire application, resulting in more efficient development and easier maintenance.
- Fast and consistent user interface design — React excels in building rich user interfaces, transcending mere aesthetics. Its components act as building blocks, enabling the creation of intuitive and visually stunning UIs. Each interaction, button, and visual element can be meticulously crafted and customized, ensuring an engaging user experience. React is a foundation of many design systems.
- Reusable components — Once you create a component, you can reuse it in multiple parts of your application without having to rewrite the same code. This reduces redundancy, making your codebase more concise and easier to maintain.
- Flexibility — With the ability to create anything from static websites and desktop applications to iOS or Android mobile apps, React adapts to diverse project requirements. This adaptability is bolstered by its extensive community, which over time has developed a myriad of tools, libraries, and extensions.
- Great user experience — React’s prowess in facilitating instant updates without reloading the entire page is a game-changer. This feature provides a smoother and faster user experience, exemplified by actions like ‘liking’ a post on Facebook, where changes occur seamlessly without the need for a full page refresh.
- Community — The sheer size and activity of React’s community further solidify its standing. With over 460,000 questions on Stack Overflow’s ‘React.js’ thread and JavaScript’s extensive support, developers can find a wealth of resources and solutions, making React an accessible and well-supported technology.
What can you build with React?
React is a versatile and popular JavaScript library that can be used to build a wide range of React projects.
React is well-suited for creating Single-Page Applications where a single HTML page is dynamically updated as the user interacts with the app. Examples include social media platforms, project management tools, and real-time collaboration apps.
React can also be used to build eCommerce sites. React’s ability to efficiently update the user interface makes it ideal for this type of project. You can create dynamic product listings, shopping carts, and seamless checkout experiences.
Just check out our pre-built eCommerce templates that we include in our tool, UXPin Merge. Those templates are perfect examples of what can be created as a React project. We have a React shopping cart, product page, and product listing. You can quickly copy them to your workflow.
React is great for building data dashboards that require real-time updates. This is particularly useful for analytics tools, monitoring systems, and business intelligence applications that need to streamline internal operations.
What’s more, React can be integrated with mapping libraries to create interactive and dynamic maps. This is useful for applications that involve geolocation, such as travel apps or location-based services. It’s also great for weather apps that utilize maps and location.
Applications that require real-time collaboration, such as messaging apps, collaborative document editing tools, learning management systems (examples), and video conferencing platforms, can benefit from React’s ability to efficiently update the user interface.
Build your app layout with React components
In this article, we explored how React works, its basic features, and give you an idea of what you can build with React components. If you want to experiment now, let’s head on to UXPin and test React by creating a simple app interface. Set up a UXPin trial account and create a new project. Choose MUIv5 library from Design System Library (use keys Option + 2 to open it) and move components onto the canvas. It’s as simple as that.
You can build whatever you want and if you need to access documentation, just click the component and see it linked on the right. Build your first React-based user interface today. Try UXPin Merge.